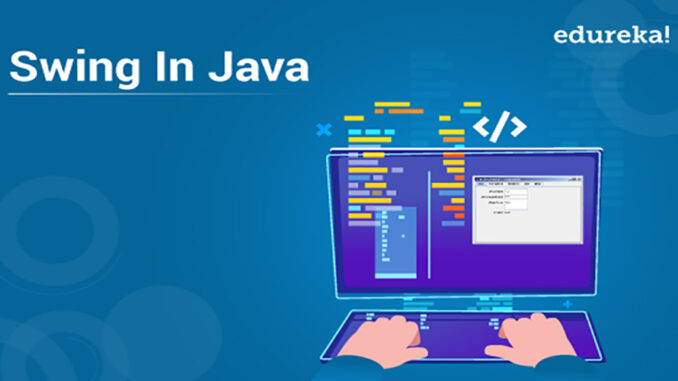
Viết chương trình Java với GUI để hiển thị các tập tin và thư mục. Khi nhấp vào một thư mục, tất cả các tệp và thư mục con sẽ được hiển thị trong giao diện người dùng.
Phần code giải:
package Practices;
import java.awt.BorderLayout;
import java.awt.DisplayMode;
import java.awt.EventQueue;
import java.awt.Panel;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.File;
import javax.swing.JButton;
import javax.swing.JFileChooser;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
import javax.swing.JTree;
import javax.swing.border.EmptyBorder;
import javax.swing.tree.DefaultMutableTreeNode;
import javax.swing.tree.DefaultTreeModel;
import javax.swing.tree.TreeModel;
import javax.swing.tree.TreePath;
public class ex1_FileBrowserGUI extends JFrame {
private static final long serialVersionUID = 1L;
private JPanel panel;
private JLabel label;
private JButton browseButton;
private JTree fileTree;
private JScrollPane scrollPane;
private JFileChooser filechooser;
public ex1_FileBrowserGUI () {
panel = new JPanel();
label = new JLabel();
browseButton = new JButton("Browse");
fileTree = new JTree();
scrollPane = new JScrollPane(fileTree);
filechooser = new JFileChooser();
browseButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
int choice = filechooser.showOpenDialog(ex1_FileBrowserGUI .this);
if(choice == JFileChooser.APPROVE_OPTION) {
File selectedFile = filechooser.getSelectedFile();
displayFiles(selectedFile);
}
}
});
panel.add(label);
panel.add(browseButton);
add(panel, BorderLayout.NORTH);
add(scrollPane, BorderLayout.CENTER);
setTitle("File Browser");
setSize(500,400);
setDefaultCloseOperation(EXIT_ON_CLOSE);
setVisible(true);
setLocationRelativeTo(null);
}
private void displayFiles (File directory){
DefaultMutableTreeNode rootNode = new DefaultMutableTreeNode(directory.getName());
DefaultMutableTreeNode treeModel = new DefaultMutableTreeNode(rootNode );
fileTree.setModel((TreeModel) treeModel);
traverseDirectory(directory, rootNode);
}
private void traverseDirectory (File directory, DefaultMutableTreeNode parentNode) {
File[] files = directory.listFiles();
if (files != null) {
for(File file : files) {
DefaultMutableTreeNode node = new DefaultMutableTreeNode(file.getName());
parentNode.add(node);
if(file.isDirectory()) {
traverseDirectory(file, node);
}
}
}
}
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
ex1_FileBrowserGUI frame = new ex1_FileBrowserGUI ();
frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
}
Đoạn mã trên là một ứng dụng GUI đơn giản để duyệt và hiển thị cấu trúc thư mục của máy tính. Dưới đây là giải thích cho từng phần của mã:
- Khai báo các thành phần giao diện người dùng:
panel
: Panel chứa các thành phần khác.label
: Nhãn để hiển thị thông báo cho người dùng.browseButton
: Nút “Browse” để chọn thư mục.fileTree
: Cây hiển thị cấu trúc thư mục.scrollPane
: Thanh cuộn để chứa cây.filechooser
: Đối tượng JFileChooser để chọn thư mục.
- Xử lý sự kiện cho nút “Browse”:
- Khi người dùng nhấp vào nút “Browse”, một hộp thoại JFileChooser được hiển thị để cho phép người dùng chọn thư mục.
- Nếu người dùng chọn một thư mục, phương thức
displayFiles()
được gọi với tham số là thư mục đã chọn để hiển thị cấu trúc thư mục trên cây.
- Phương thức
displayFiles(File directory)
:- Tạo một nút gốc (rootNode) từ tên thư mục được chọn.
- Tạo một cây mới với nút gốc và đặt nó làm mô hình của cây.
- Gọi phương thức
traverseDirectory()
để duyệt qua các tệp và thư mục trong thư mục đã chọn và thêm chúng vào cây.
- Phương thức
traverseDirectory(File directory, DefaultMutableTreeNode parentNode)
:- Lấy danh sách các tệp và thư mục trong thư mục đã cho.
- Duyệt qua danh sách và tạo một nút cho mỗi tệp hoặc thư mục.
- Nếu tệp hoặc thư mục là một thư mục, gọi đệ quy phương thức
traverseDirectory()
để duyệt qua các tệp và thư mục bên trong và thêm chúng vào nút hiện tại.
- Phương thức
main()
:- Tạo một đối tượng của lớp
ex1_FileBrowserGUI
và hiển thị giao diện người dùng.
- Tạo một đối tượng của lớp
Ứng dụng này tạo một cửa sổ GUI với một nút “Browse”. Khi người dùng nhấp vào nút, hộp thoại chọn thư mục xuất hiện và người dùng có thể chọn một thư mục từ đó. Sau đó, cây sẽ hiển thị cấu trúc thư mục của thư mục được chọn.
THANKS FOR YOUR TIME !!!
Để lại một phản hồi
Bạn phải đăng nhập để gửi phản hồi.